I am a backend engineer and we are working on a new Linux Project. We have UI UX team with us which they mainly work on UI UX front end. Once they are done with their UI UX work, they handed it over to us – backend engineers to integrate UI UX stuff with the backend Linux daemon service which runs in the background and/or at startup. After the integration, we found few issues with UI UX stuff mainly filtering as we have many options for end user to filter in the front end and I was also assigned to fix them which I did. Through working on fixing them, I came across JS code which uses IndexOf to find a match and I would have thought IndexOf(“”) empty string should yield -1 but it does not and instead it yields 0 and I also wonder what would be yielded in C#. I did a quick console app in .NET to test. And as a result, C# behaves the same way – returns 0.
From MSDN :
https://docs.microsoft.com/en-us/dotnet/api/system.string.indexof?view=net-5.0
String.IndexOf Method
Definition
Namespace:SystemAssemblies:mscorlib.dll, System.Runtime.dll
Reports the zero-based index of the first occurrence of a specified Unicode character or string within this instance. The method returns -1 if the character or string is not found in this instance.
But not too quick, if we go further below here it stated:
Returns
The zero-based index position of value
from the start of the current instance if that string is found, or -1 if it is not. If value
is Empty, the return value is startIndex
.

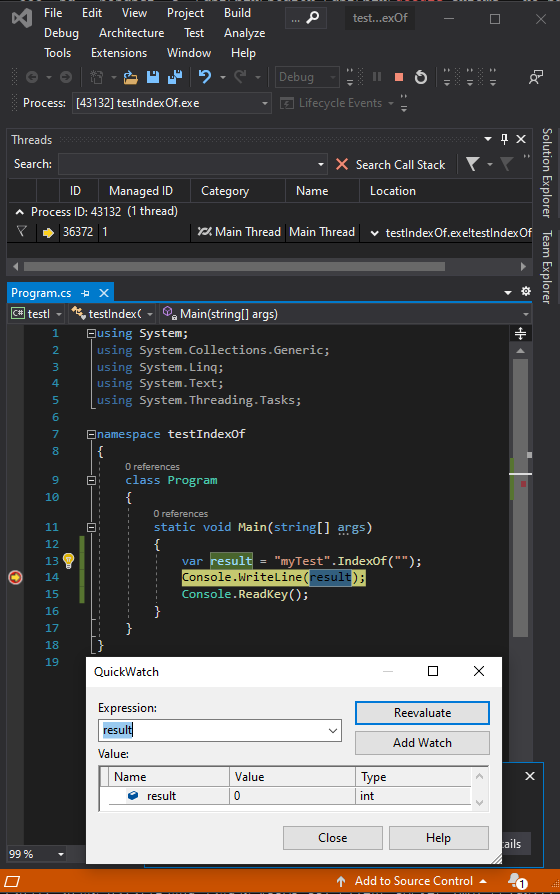